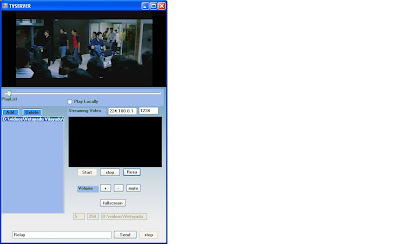
TV CLIENT
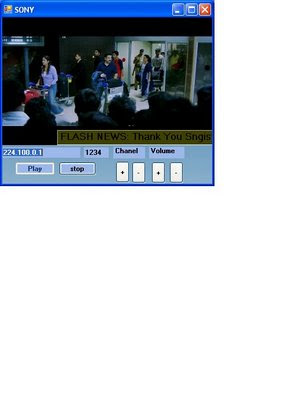
With the help of Video Lan Player we can easily stream video over a lan using c#.net.For This Project You must do the following steps.
Step 1:
Install vlc player in your computer.For more information about vlc player visit http://www.videolan.org/.
Step 2:
Just copy the libvlc.dll from the vlc installaling folder and paste it in to the windows->system32 folder.
Step 3:
Create a class named LibVlc.cs.You will get the Source code of LibVlc.cs from http://libvlc.blogspot.com/.
Step4:
Server Side
You design a form contain a picture box and a button.Just copy paste the source code in the button click event.
private void button1_Click(object sender, EventArgs e){
try
{
LibVlc vlc = new LibVlc();
vlc.Initialize();
vlc.VideoOutput = pictureBox1;
vlc.PlaylistClear();
string[] Options=new string[] { ":sout=#duplicate{dst=display,dst=std {access=udp,mux=ts,dst=224.100.0.1:1234}}" };
vlc.AddTarget("c:\\1.flv", Options);
vlc.Play();
}
catch (Exception e1)
{
MessageBox.Show(e1.ToString());
}
}
Step 5:
Client Side:
In client side we can play video from a stream produced by the server.Design a form contain a picture box and a button.Just copy paste the code in the button click event.
private void button1_Click(object sender, EventArgs e){
try
{
LibVlc vlc = new LibVlc();
vlc.Initialize();
vlc.VideoOutput = pictureBox1;
vlc.PlaylistClear();
string[] options = { ":sout=#duplicate{dst=display,dst=std{access=file,mux=asf,dst=\"F:\\My-Output-Video-Filename.asf\"}}" };
vlc.AddTarget("udp://@224.100.0.1:1234", options);
vlc.Play();
}
catch (Exception e1)
{
MessageBox.Show(e1.ToString());
}
}
Code Explanation:
Server Side:
In server side first we create the object of the class LibVlc.cs created in step3.
Set the vlc.VideoOutput=our picture box name.
In vlc.AddTarget() method we pass two para metres.First one is the full path of the file name to be streamed.Second one is the string array contain the protocol type,address and a port number.Here protocol type is udp.
224.100.0.1-multi cast ipaddress.Also use unicast ip address or a loop back address(127.0.0.1) when you use a single system.
If you want to know more about multicast and unicast ipaddress vist http://unicastandmulticast.blogspot.com/.
1234-port number.If you want to know more about multicast and unicast ipaddress vist http://unicastandmulticast.blogspot.com/.
When the vlc.play() method is executed,Video is playing locally and stream the video over the Lan at the same time.
Client Side:In client side first four lines as same as the client side.In vlc.Addtarget() method we pass two parameters first one contain protocol type ipaddress and the port number.Second one is a string array.After this method we can call a method vlc.play().Then video is playing in our picture box from the Network stream.
We can easily make television broadcasting stations in the local lan in c#.net.I done a project using this codes view the screen shots of that project.http://videostreamscreenshots.blogspot.com/
Note:I use vlc media player 0.8.6i.
About MeArun Sukumar
hit counter |
61 comments:
Hi Arun,
I am facing some problem in following line of code. Do you have any suggestions?
string[] Options = new string[] { ":sout=#duplicate{dst=display,dst=std {access=file,mux=raw,dst=\"D:\\sms.mpg\"}}" };
vlc.AddTarget("D:\\nn.bin", Options);
vlc.Play();
Hi Arun,
I am facing some problem in following line of code. Do you have any suggestions?
string[] Options = new string[] { ":sout=#duplicate{dst=display,dst=std {access=file,mux=raw,dst=\"D:\\sms.mpg\"}}" };
vlc.AddTarget("D:\\nn.bin", Options);
vlc.Play();
Hello Arun,
There is no error in the code, but nothing is bieng played... What am i suppossed to change to make it work it properly...
Akz
Hai Akz if ur pc not connected with
a network.pls use 127.0.0.1 insted of
224.100.0.1 and set the correct path of the video.don't work pls mail me.
mail id arunsuku4u@gmail.com
Hi Arun,
I tried to debug through the code and encounter this error.
"Could not find libvlc"
I have already paste the libvlc.dll into system32 drive.
Any idea?
Thanks in advance.
Hi Arun,
I tried to debug through the code and encounter this error.
"Could not find libvlc"
I have already paste the libvlc.dll into system32 drive.
Any idea?
Thanks in advance.
plz send the answer to harish.puramsetti@gmail.com
hi Arun,
I am facing problems while debugging, it says System.AccessVoilationException: Attempted to read or write protected memory...
how to handle this..??
Hello, I just want to big thanks for your sharing.I really like the ideas, simple player but meaningful. Please how can I write codes for Volume (+-), Add, Delete, Resume,Full Screen, Mute and Relay?
Which Version of Visual Studio are U using? I have problem in using Microsoft.Win32, I am using VS 2005
Microsoft.Win32 is solved
I am facing the problem of streaming between two computers. on the server side .flv file is running well in the picture box. but i connected it with another laptop as client side, but i am not able to see any output. one file by the name of My-Output-Video-Filename.asf is made but it is of 0 bytes and nothing is shown in the picture box..
The problem of SystemAccessViolation is solved. It was coming because of vlc.VideoOutput = pictureBox1;
after removing this line problem is solved..
HelloVishu, The pictureBox didn't make any impact bcos when I click on server it open seperate window and same to client side.
Why I can not to another PC? I have 224.100.0.1 and even I tried to use Server IP (192.168.0.140) and I can not receive streaming at the Client IP (192.168.0.150)
even i tried using different addresses for server and clients.
but its not even working for unicast case.. i tried using 127.0.0.1:1234
on both form1 i.e. server and form2 i.e. client, on my laptop only and added two lines in form1:
Form2 ff = new Form2();
ff.Show();
still am not getting anything on the client side.. movie is running well in case of server side only...
Hello Vishu,
Same to me I havn't get it working. I even regsvr32 the vlc on server and client PCs but all the same. Alright now I am checking VLC forum site for solutions. Let keep trying and Arun did not respond to his blog.
hello AfriTelly,
tell me one more thing, as you said its not working in case of the IP address you mentioned, but have you tried it for unicast, mine is not even working for unicast case, do tell me if it works, and also the procedure how you made it run..?
Hello vishu and Afritelli thank for ur comments.pls send your problems in my mail.arunsuku4u@gmail.com.I will try to help you.
Alright, I will send it to ur email now
HelloArun,
I have replied you by email.
Hello Vishu,
Look at this diagram of modules on VLC.
This is the link http://www.videolan.org/developers/vlc/doc/doxygen/html/group__libvlc.html
Observation about VLC streaming over LAN. Hows to pass behinde firewall, use 12. 12 is the value of the TTL (Time To Live) of your IP packets (which means that the stream will be able to cross 11 routers).
hi arun,
very good work, what i want to know is how to extend this for the video streaming across the internet
Hi Sukumar
First Thanks for placing the code in this site
I have used ur code and when try to run it i got the error "Unable to find the libvlc.dll though i have placed it in System32"
Can u please resovle this for me
Thanks in advance
Harikrishna.k
ph:9052939303
Mail:kodaliharikrishna@gmail.com
Hi, Arun.
Your code doesnt work, Can you send me complated code to my email h_yunusov@mail.ru Thanks
HI ARUN
I HAVE A PROBLEM WITH THE NEXT FUNCTION
private string QueryVlcInstallPath()
{// open registry
RegistryKey regkeyVlcInstallPathKey = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\VideoLAN\VLC"); HERE I INDICATED, WHERE I INSTALLED THE VLC?...........
if(regkeyVlcInstallPathKey == null)
return "";
return (string)regkeyVlcInstallPathKey.GetValue("InstallDir","");
}
AND IN THE NEXT FUNCTION
public bool Initialize()
{// check if already initializes
if(m_iVlcHandle != -1)
return true;
// try init
try
{
// create instance
m_iVlcHandle = VLC_Create();
if (m_iVlcHandle < 0)
{
m_strLastError = "Failed to create VLC instance";
return false;
}
// make init optinons
string[] strInitOptions = { "vlc",
"--no-one-instance",
"--no-loop",
"--no-drop-late-frames",
"--disable-screensaver"};
if(m_strVlcInstallDir.Length > 0)
strInitOptions[0] = m_strVlcInstallDir + @"\vlc"(HERE I INDICASTED THE LIBRARY LIBVLC ?);
// init libvlc
Error errVlcLib = VLC_Init(m_iVlcHandle, strInitOptions.Length, strInitOptions);
if (errVlcLib != Error.Success)
{
VLC_Destroy(m_iVlcHandle);
m_strLastError = "Failed to initialise VLC";
m_iVlcHandle = -1;
return false;
}
}
catch
{
m_strLastError = "Could not find libvlc";
return false;
} HERE ENCOUNTER THIS ERROR: "Could not find libvlc"
AND "excepcion HRESULT:0x8007007e"
// check output window
if(m_wndOutput != null)
{
SetVariable("drawable",m_wndOutput.Handle.ToInt32());
wndOutput_Resize(null,null);
}
// OK
return true;
}
PLEASE HELP ME,
THANK VERY MUCH…..
hi ,
this code runs fine.. but while receiving live streams i am getting an error stating "Libvlc has encountered a problem and needs to close" .. Can u please help on this?? i want it to receive live streams without any problems inbetween..
hi,
will this code work only for certain versions of vlc.. When executing the ecode with with vlc0.8.6 it works fine. however with vlc 1.0.5 its not displaying anything
Could you Send this project to ma mail?
Hi Arun This is Aryan Dev.
i copy-paste code avable at http://libvlc.blogspot.com/.
save it as "LibVlc.cs" in Current Project but During compling it Show Error At
=================
if (m_iVlcHandle < m_strlasterror = "Failed to create VLC instance" strinitoptions =" {"> 0)
strInitOptions[0] = m_strVlcInstallDir + @"\vlc";
==================
of Excepted "(" and ";" hey i m New to C#.net and not getting wat to write in betten
create VLC instance" strinitoptions =" {"> 0)
Pls Help me out me Sending u mail regarding this Problem also...
thanks in advance...for both sharing code and helpig me Ya can u provide same code in .zip format than their will b less chance to generating error Thanks... again boss...
-Aryan dev
Hi,
I have a problem with the libvlc.dll.
I already placed it in the system32 folder but it still does not detect it.
Thank you very much
hi Kadhambari
can you share your code with me. working with vlc 0.8.6
mail me at : sanjudusad at gmail
please/ i need help/
pls send you project to mak.sum@mail.ru
in my version(vlc 1.1.0) picturebox is empty
There is no error in the code, nothing playing in picturebox.video path is correct.can u give a suggesstion.
or Send ur sample project,
sacsrini@gmail.com
// try init
try
{
// create instance
m_iVlcHandle = VLC_Create();
if (m_iVlcHandle < m_strLastError = "Failed to create VLC instance"
strinitoptions =" {"> 0;);
strInitOptions[0] = m_strVlcInstallDir + @"\vlc";
init libvlc
Error errVlcLib = VLC_Init(m_iVlcHandle, strInitOptions.Length, strInitOptions);
if (errVlcLib != Error.Success)
{
VLC_Destroy(m_iVlcHandle);
m_strLastError = "Failed to initialise VLC";
m_iVlcHandle = -1;
return false;
}
}
i have problem with this lines, plz help me, thx so much
the If statement at the first line is not correct? is it?
I get 2 errors using C# 2010
1. LibVlc is a namespace but is used like a type
code:
try
{
LibVlc vlc = new LibVlc();
vlc.Initialize();
vlc.VideoOutput = pictureBox1;
vlc.PlaylistClear();
string[] Options = new string[] { ":sout=#transcode{vcodec=h264,vb=800,scale=1,acodec=mp4a,ab=128,channels=2,samplerate=44100}:duplicate{dst=rtp{dst=10.10.11.238,port=1234,mux=ts},dst=display}" };
vlc.AddTarget("c:\\Planet.avi", Options);
vlc.Play();
}
catch (Exception e1)
{
MessageBox.Show(e1.ToString());
}
Can someone please assist ?
Thank you!
Regards
Jaco
Jaco,
You should use a "using" directive to tell the compiler what's the namespace of the LibVlc class.
If you're working in Visual Studio, stand with the cursor on the "LibVlc" class name. You'll see a little rectangle at the bottom right of the class name. Click it and select the "using" option.
Hello Arun,
I cannot get the code to work with the following setup:
1 ) my server is a VLC player that is streaming a udp H.264 video with the following output string:
:sout=#transcode{vcodec=h264,vb=0,scale=0,acodec=mp4a,ab=128,channels=2,samplerate=44100}:udp{dst=224.0.0.1:1234} :no-sout-rtp-sap :no-sout-standard-sap :sout-keep
2) my client is the same as the one posted on this blog with nothing is playing ... why ? any ideas ?
Hello Again,
now im using your server but it not play/stream the video. i think it is the way my VLC is setup. any ideas ?
the way i setup my project is by installing VLC , then copy-paste libvlc.dll to system 32 and got the Libvlc.cs . i just followed you example but nothing happened when i launch the server.
Thanks
pls to send stream video over a lan using c#.net exe files ........... didnt send exe files..... to change the or delete . and exe files extension(ex:lib.exe)to change (lib)to send sir....
Hi Arun,
I am facing the same problem as many have shared.There is an exception thrown at
m_iVlcHandle = VLC_Create();
The exception says
Unable to load DLL 'libvlc': The specified module could not be found. (Exception from HRESULT: 0x8007007E)
But i already pasted libvlc in System32
Lebna, I had the same problem, just write to environment variables vlc and vlc/plugins path
Aygun,
Thanks a lot!!! I am really sorry but could you please tell me how to write to the environment variable and the plugin path.
Thanks a lot for the help!!
i have compiled the server side code and LibVlc.cs file together in a project...but its giving error..in the following line..
if (m_iVlcHandle < m_strlasterror = "Failed to create VLC instance" strinitoptions =" {"> 0)
and also d error saying LibVlc is a namespace and connot be used as a type..in...
LibVlc vlc = new LibVlc();
plz help...i am in desperate need of this project...
hi arun,
the code is not working 4 me..plz try 2 send me the corrected code at mittal.ankit19@gmail.com
i have compiled the server side code and LibVlc.cs file together in a project...but its giving error..in the following line..
if (m_iVlcHandle < m_strlasterror = "Failed to create VLC instance" strinitoptions =" {"> 0)
and also d error saying LibVlc is a namespace and connot be used as a type..in...
LibVlc vlc = new LibVlc();
plz help..
mail me the solution at mittal.ankit19@gmail.com
i need pass a stream as target instead of a file and i don´t know if it is possible
is it possible to do this project using wcf?
Hi Arun,
I am facing problem in following line code (vs2005).
Do you have any suggestion?
// create instance
m_iVlcHandle = VLC_Create();
if (m_iVlcHandle < m_strlasterror = "Failed to create VLC instance" strinitoptions =" {"> 0)
strInitOptions[0] = m_strVlcInstallDir + @"\vlc";
I have compiled the server side code and LibVlc.cs file together in a project...but its giving error..in the following line..
if (m_iVlcHandle < m_strlasterror = "Failed to create VLC instance" strinitoptions =" {"> 0)
and also the error saying LibVlc is a namespace and connot be used as a type..in...
LibVlc vlc = new LibVlc();
This is URGENT!!!!!!!!
please mail me the solution ASAP at vishalnoronha14@gmail.com
it's not working. the picturebox don't show anything. pls help me.
Hi Arun
can u tell me How to work these functions SpeedFaster() SpeedSlower().
coz i am using these function in my code its not working...
Hi Arun,
I tried to debug through the code and encounter this error.
"Could not find libvlc"
I have already paste the libvlc.dll into system32 drive.
Any idea?
please revert me back on swapnil_vengurlekar@yahoo.com
having problem running the code. the video is not running on the server side nor the client side. what changer should i make
Wonderful blog! I never ever read such kind of information that imparted me great knowledge.
best tv box
best android tv box
Can we stream a usb cam locally and record as per requirement, like in vlc application we can stream video
Borgata - The Sports Book - Worrione
The Sports Book at Borgata! Borgata Hotel Casino & Spa is the premier Integrated Sportsbook in Atlantic City and features over 1,000 games on 바카라 사이트 온라인 바카라 various
Harrah's Atlantic City - MapYRO
Find 아산 출장마사지 all Harrah's Atlantic City hotels and motels in one 정읍 출장샵 place. Earn reward points 구미 출장안마 for nonstop fun at Harrah's. 광주 출장샵 Earn Harrah's casino 서울특별 출장마사지 bonus credits when you
Post a Comment